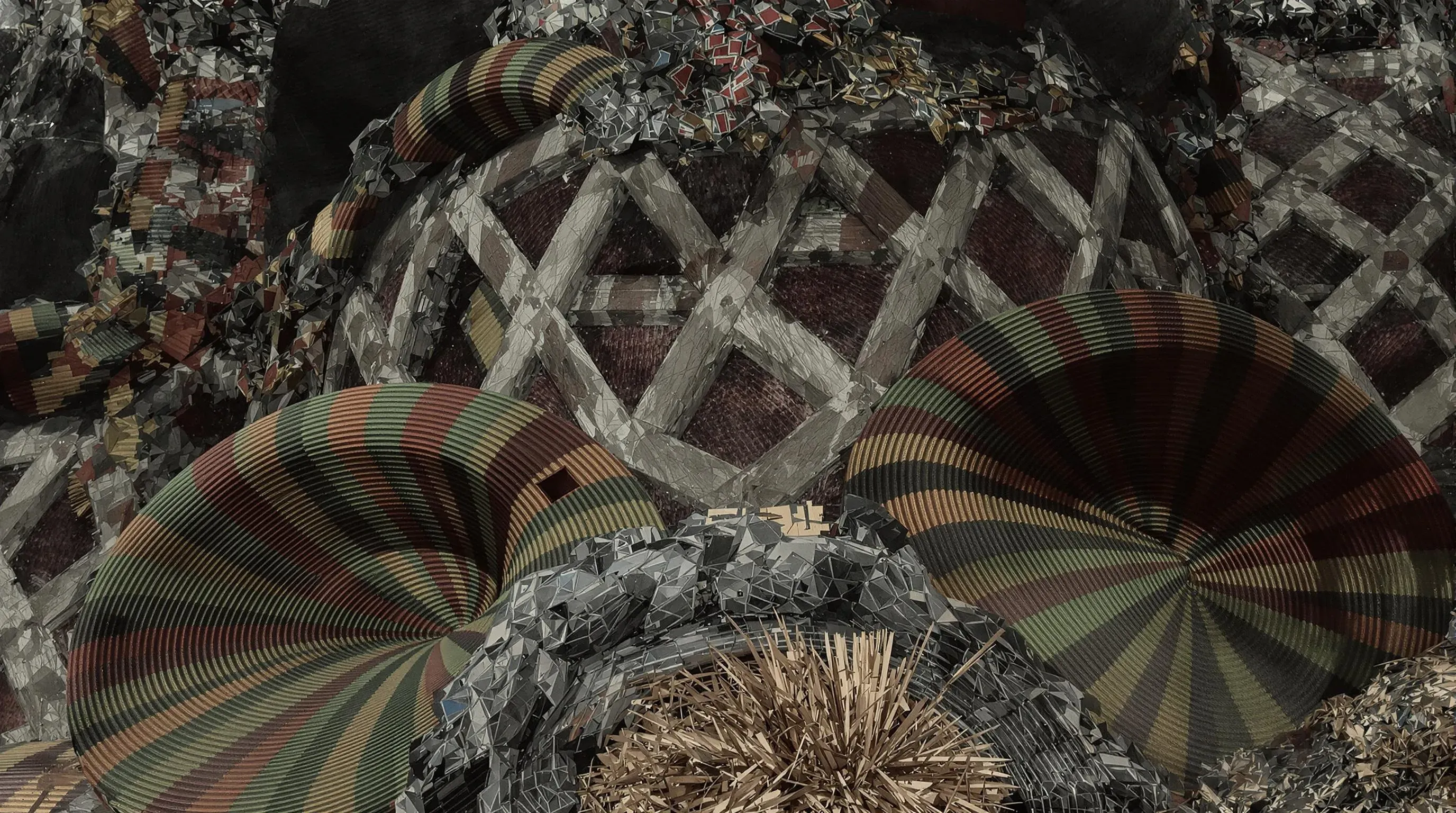
#28.1 Part 1/3 Basics of Software Architecture and Design Patterns
/ 4 min read
Updated:Why these patterns matter?
Modern software development faces constant challenges. As our applications grow bigger and more complex, we need smart ways to keep our code clean and manageable. Design patterns help us solve these challenges.
Think of design patterns as battle-tested blueprints for solving common programming problems. They’re like recipes that have been refined by countless developers over many years. When you use these patterns, you’re not just writing code – you’re applying solutions that are proven to work.
These patterns help us write better software by:
- Making our code easier to maintain
- Helping teams work together more effectively
- Making it simpler to add new features
- Keeping our applications scalable
By learning and using design patterns, you’re not reinventing the wheel – you’re building on the experience of the entire development community.
Why Are Design Patterns Important? (The Details)
- Code Reusability: Design patterns promote modular and reusable code, reducing redundancy and improving efficiency. By using patterns, developers can avoid reinventing the wheel and apply pre-tested solutions to their software projects.
- Scalability and Maintainability: Well-structured applications are easier to maintain and extend. Design patterns ensure that software is scalable, allowing new features to be integrated without major refactoring.
- Improved Collaboration: Using common patterns allows teams to communicate effectively. Instead of explaining complex logic, developers can refer to a known pattern, making collaboration across teams easier.
- Code Readability and Structure: By following design patterns, developers ensure clearer, more structured code, making it easier for others (or even their future selves) to understand and modify.
- To Solve Recurring Problems: Many software design challenges are common across different projects. Design patterns provide structured solutions that have been tested and refined over time.
- To Reduce Development Time: Using design patterns accelerates development by eliminating trial-and-error approaches. Developers can focus on building new features rather than solving architectural issues from scratch.
- To Improve Code Consistency: Patterns enforce standardization in coding practices, making software more predictable and reducing the risk of inconsistent implementations.
When Should Design Patterns Be Used?
Design patterns do help a lot building the basis of a projectstructure. BUT in some cases it is just not necessary to use them. For example: Using the finest materials on a ferrarri (trademark: ferrarri) makes sense. Using the finest materials on a family van not so much. So when should you use design patterns?
🔹 Use Design Patterns When:
- The project is large and complex.
- The same problem reoccurs across multiple projects.
- The codebase needs to be maintainable and extendable.
- Development involves multiple teams and requires standardization.
- There is a need for scalability without massive code rewrites.
❌ Avoid Overusing Design Patterns When:
- The project is small and simple.
- The pattern introduces unnecessary complexity.
- It is used just for the sake of using it, without solving a real problem.
How to Start Implementing Design Patterns?
1️⃣ Learn the Basic Categories
Design patterns are divided into three main categories:
- Creational Patterns – Managing object creation (e.g., Singleton, Factory Method, Builder).
- Structural Patterns – Organizing code structure (e.g., Adapter, Composite, Facade).
- Behavioral Patterns – Managing communication between objects (e.g., Observer, Strategy, Command).
2️⃣ Start with Commonly Used Patterns
Begin with patterns that are widely used and easy to understand:
- Singleton Pattern – Ensures a class has only one instance.
- Factory Method – Provides a way to create objects without specifying the exact class.
- Observer Pattern – Implements a subscription-based model.
- Strategy Pattern – Enables swapping different algorithms at runtime.
3️⃣ Apply Patterns in Small Projects
Instead of diving into a large-scale implementation, start by using patterns in small personal projects or refactoring existing code.
4️⃣ Use Design Patterns in Real-World Scenarios
- Implement Factory Pattern when dealing with different types of database connections.
- Use Observer Pattern for handling event-driven applications.
- Apply Facade Pattern to simplify interaction with third-party APIs.
5️⃣ Read and Practice
- Read “Design Patterns: Elements of Reusable Object-Oriented Software” (by the Gang of Four).
- Explore open-source projects and observe how patterns are used.
- Practice implementing patterns in different programming languages.
Conclusion
Design patterns are essential tools for modern software development. By understanding and applying these patterns, developers can build scalable, maintainable, and efficient applications. Whether you’re working on a personal project or a large enterprise application, design patterns provide a solid foundation for success.
In the next part, we will dive deeper into the different types and principles of design patterns and how they can be used in your projects. Stay tuned!
Any Questions?
Contact me on any of my communication channels: