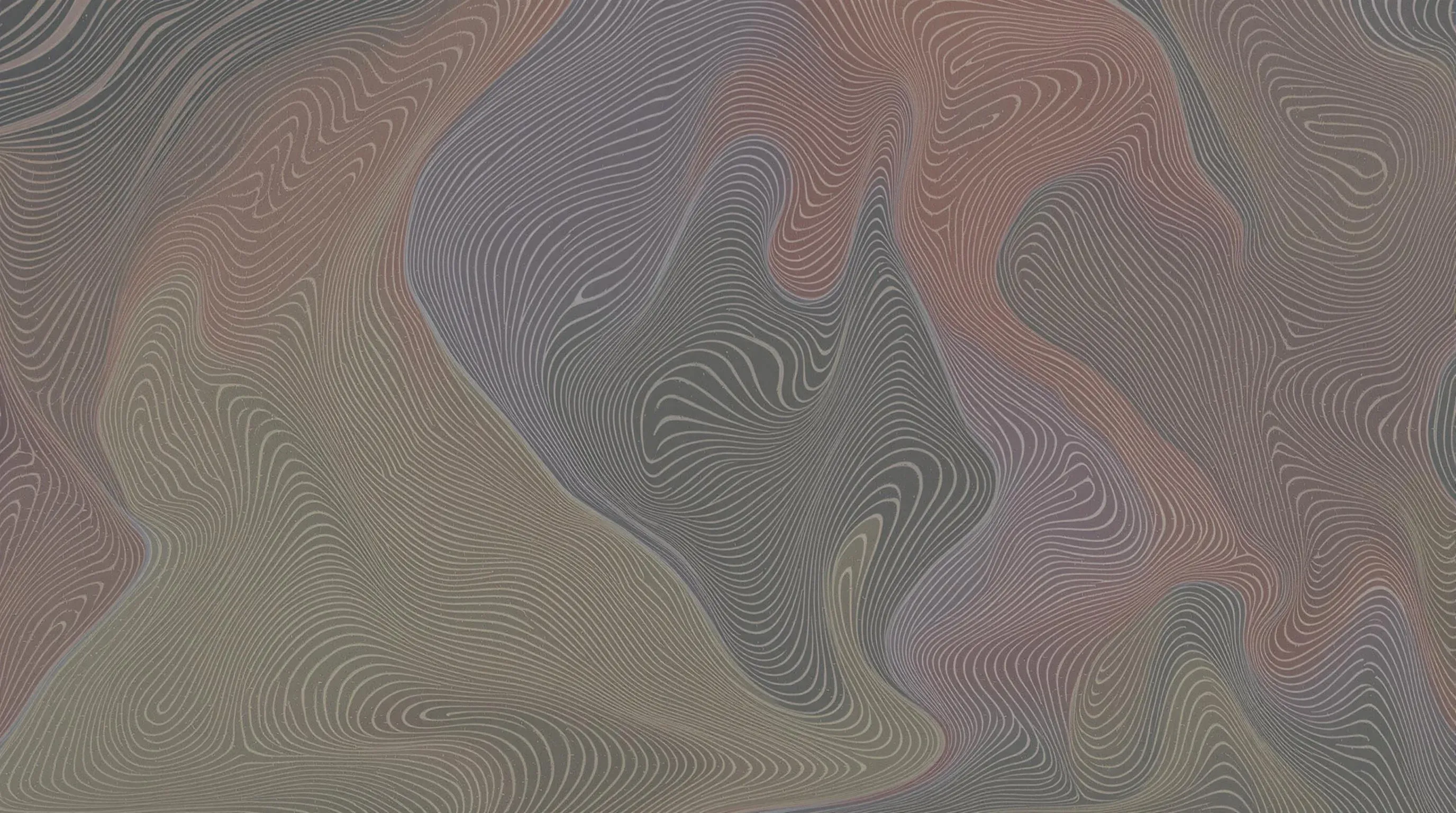
#28.2 Part 2/3 Basics of Software Architecture and Design Patterns
/ 5 min read
Updated:The Power of Design Patterns in Software Development
Intro 2
Last time we spoke about all the benefits and reasons why design patterns are important. Today we will dive into the different types and principles of design patterns and how they can be used in your projects.
Aspects of Software Architecture
1. Modularity
Modularity is the practice of dividing a system into smaller, independent modules, each responsible for a specific functionality. These modules can be developed, tested, and maintained independently.
- Enhances maintainability by making it easier to locate and fix issues.
- Increases reusability, allowing modules to be used in different applications.
- Facilitates parallel development, enabling different teams to work on separate modules.
Microservices architecture exemplifies modularity, where each microservice represents an independent module that handles a distinct business function, such as authentication, payment processing, or inventory management.
2. Separation of Concerns (SoC)
Separation of Concerns (SoC) is a design principle that ensures different parts of a system handle distinct functionalities without unnecessary dependencies.
- Simplifies development and debugging by isolating functionalities.
- Reduces code complexity and enhances scalability.
- Enables teams to work on different aspects of a system independently.
The Model-View-Controller (MVC) pattern demonstrates SoC by dividing a software application into:
- Model: Handles data and business logic.
- View: Manages user interface and presentation.
- Controller: Processes user input and updates the model or view accordingly.
3. Single Responsibility Principle (SRP)
SRP states that each class, module, or function should have only one reason to change by being responsible for a single task or functionality.
- Reduces system complexity.
- Improves code maintainability and flexibility.
- Prevents unintended side effects when modifying code.
A UserAuthenticationService class should only handle user authentication, while a DatabaseService should manage database operations. This ensures each service has a single responsibility.
4. Scalability
Scalability is the ability of a system to handle increasing loads efficiently, either by adding more resources (horizontal scaling) or upgrading existing resources (vertical scaling).
- Ensures system performance remains stable as demand increases.
- Reduces downtime and improves user experience.
- Supports business growth without major architectural changes.
A load balancer distributes requests among multiple servers to achieve horizontal scaling, preventing any single server from becoming a bottleneck.
5. Performance Optimization
Performance optimization involves designing a system to maximize efficiency while minimizing resource consumption.
-
Enhances user experience by reducing latency.
-
Improves system efficiency and reduces operational costs.
-
Ensures smooth handling of high traffic loads.
-
Implementing caching mechanisms (e.g., Redis, Memcached).
-
Using efficient algorithms and data structures.
-
Optimizing database queries with indexing and proper schema design.
Using a Content Delivery Network (CDN) to cache and serve static assets closer to users significantly improves page load times.
6. High Availability & Fault Tolerance
High availability ensures a system remains operational despite failures, while fault tolerance allows the system to continue functioning with minimal disruption.
-
Prevents revenue loss and maintains customer trust.
-
Ensures business continuity.
-
Reduces downtime and operational risks.
-
Redundant servers and database replication.
-
Automated failover mechanisms.
-
Distributed systems with load balancing.
Cloud-based architectures with auto-healing instances detect failures and automatically replace malfunctioning components.
7. Security
Security in software architecture protects against unauthorized access, data breaches, and vulnerabilities.
-
Ensures data integrity and confidentiality.
-
Builds user trust and compliance with regulations (e.g., GDPR, HIPAA).
-
Prevents financial and reputational damage.
-
Implement authentication & authorization mechanisms (OAuth, JWT).
-
Use encryption (TLS for communication, AES for data storage).
-
Follow secure coding principles (e.g., input validation, avoiding SQL injection).
Role-Based Access Control (RBAC) ensures that only authorized users can access certain resources.
8. Loose Coupling & High Cohesion
Loose coupling minimizes dependencies between components, while high cohesion ensures each module focuses on a well-defined purpose.
- Enhances flexibility and maintainability.
- Simplifies debugging and testing.
- Encourages modular and reusable components.
Message queues (e.g., RabbitMQ, Kafka) enable asynchronous communication between microservices, reducing direct dependencies.
9. Maintainability & Extensibility
Maintainability ensures ease of modification and debugging, while extensibility allows adding new features without disrupting existing functionality.
-
Reduces technical debt and long-term costs.
-
Enables continuous system evolution.
-
Improves developer productivity.
-
Writing modular and well-documented code.
-
Adopting design patterns like Factory, Singleton, and Dependency Injection.
A plug-in architecture allows developers to extend system functionality without modifying the core codebase.
10. Consistency & Data Integrity
Ensuring data remains accurate and consistent across different parts of a system.
-
Prevents data corruption and anomalies.
-
Maintains system reliability and trustworthiness.
-
Strong Consistency: Immediate data synchronization (ACID transactions in SQL databases).
-
Eventual Consistency: Slight delay in data propagation (NoSQL databases like DynamoDB).
Using database transactions ensures an order is not processed unless payment is successfully received.
11. Observability & Monitoring
Observability enables tracking system performance and identifying issues.
-
Helps in proactive troubleshooting.
-
Improves system reliability and uptime.
-
Logging (ELK Stack, Grafana).
-
Monitoring (Prometheus, Datadog).
Structured logging allows quick debugging by providing meaningful error messages.
12. API-First Design
Designing APIs before developing system components to ensure seamless integrations.
-
Facilitates third-party integrations.
-
Ensures consistency across microservices.
-
Use RESTful or GraphQL APIs.
-
Implement API versioning.
Designing an API contract using OpenAPI before implementing backend logic.
13. Event-Driven Architecture
Building systems that respond to real-time events instead of synchronous calls.
- Enhances responsiveness.
- Supports scalable, loosely coupled components.
Apache Kafka enables real-time processing of user interactions in applications.
14. Dependency Management
Efficiently managing libraries and external dependencies.
-
Prevents software bloat and vulnerabilities.
-
Ensures compatibility and security.
-
Use dependency injection.
-
Keep libraries updated.
Using Maven or npm for dependency management.
15. Cost Efficiency
Optimizing resources to balance performance and expenses.
- Reduces operational costs.
- Ensures sustainability.
AWS Lambda allows serverless computing, reducing infrastructure costs.
Conclusion
Software architecture encompasses various aspects, from modularity and scalability to security and performance optimization. By following architectural principles and design patterns, developers can build robust, maintainable, and scalable systems that meet business requirements and user expectations.
In the next part, we will explore the different types of design patterns and how they can be applied to solve common software design challenges.
Any Questions?
Contact me on any of my communication channels: