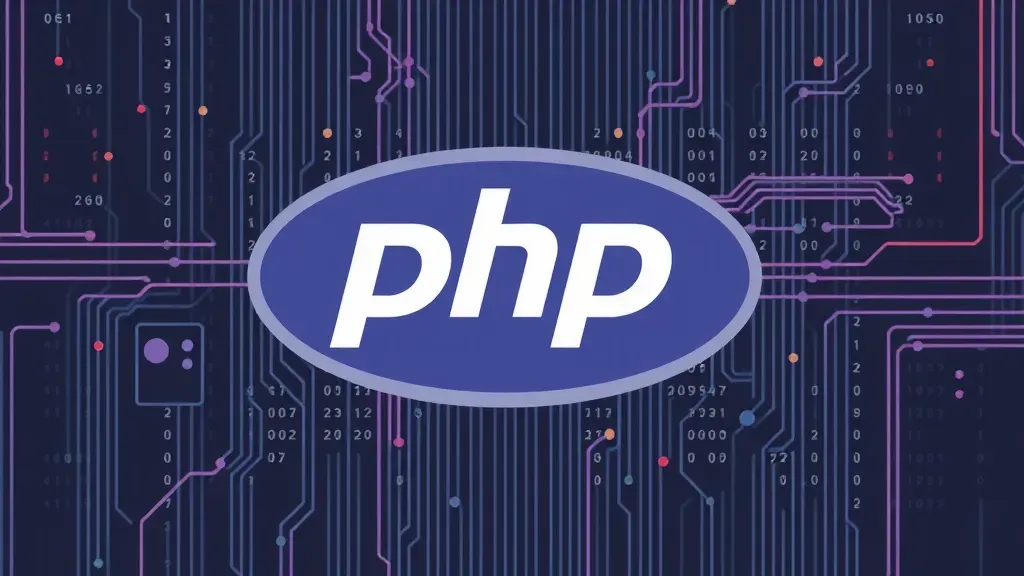
#32 PHP Server Tools for your file management needs on the server
/ 12 min read
Updated:Introduction
Server administrators and developers frequently need to perform file management operations such as extracting ZIP archives and deleting files or directories. These operations, traditionally performed via command-line interfaces or FTP clients, can be streamlined through browser-based PHP utilities. This article presents a technical analysis of three PHP scripts designed to handle common file management tasks:
extract-zip.php
- For extracting ZIP archivesdelete-folder.php
- For selectively removing directoriesdelete-script.php
- For unified management of both files and folders
We’ll examine the architecture, security implementations, and technical advantages of each tool, while providing code analysis and potential implementation scenarios.
Accessing the Tools
These PHP scripts function as standalone web applications that can be accessed through any browser. After uploading the scripts to your server:
http://example-domain.com/extract-zip.phphttp://example-domain.com/delete-folder.phphttp://example-domain.com/delete-script.php
For local development environments, you can access them via:
http://localhost/extract-zip.phphttp://localhost/delete-folder.phphttp://localhost/delete-script.php
The scripts are entirely self-contained, requiring no additional setup or configuration files to function. Simply access the URL, and the web interface will load, providing immediate access to the file management tools.
Technical Overview
All three scripts follow a similar architectural pattern:
- Configuration Layer: Setting execution parameters and defining base directories
- Functional Layer: Core file operation functions with error handling
- Request Processing Layer: Capturing and validating user input
- Response Layer: Generating human-readable feedback and UI elements
The scripts also implement critical protection mechanisms including path traversal prevention, execution time management, and confirmation steps to prevent accidental data loss.
Script 1: ZIP File Extraction (extract-zip.php
)
Technical Architecture
extract-zip.php
implements a web interface for the ZipArchive
PHP class. The script:
- Sets an extended execution time limit to handle large archives
- Scans the current directory for ZIP files
- Provides a selection interface for users
- Extracts the selected archive with detailed error handling
- Provides feedback on the extraction process
Code Analysis
1. Execution Configuration
// Set max execution time to 5 minutes (300 seconds)set_time_limit(300);
This configuration prevents script timeout during extraction of large archives. For production environments with significant ZIP processing requirements, this parameter may need adjustment based on server capabilities and expected file sizes.
2. ZIP File Discovery
function getZipFiles() { $zipFiles = []; $dir = dirname(__FILE__); if ($handle = opendir($dir)) { while (false !== ($entry = readdir($handle))) { if ($entry != "." && $entry != ".." && pathinfo($entry, PATHINFO_EXTENSION) === 'zip') { $zipFiles[] = $entry; } } closedir($handle); } return $zipFiles;}
Technical notes:
- Uses
dirname(__FILE__)
to ensure consistent path resolution regardless of execution context - Leverages
pathinfo()
withPATHINFO_EXTENSION
parameter for reliable file type identification - Explicitly excludes directory entries (
.
and..
) - Properly closes directory handles to prevent resource leaks
- Returns an indexed array for direct iteration in the presentation layer
3. Archive Extraction Engine
function extractZip($zipFile) { // Create a ZIP archive object $zip = new ZipArchive();
// Open the ZIP file $result = $zip->open($zipFile);
$extractedFiles = [];
if ($result === TRUE) { // Get current directory (where this script and ZIP file are located) $extractPath = dirname(__FILE__);
// Extract the contents of the ZIP file $zip->extractTo($extractPath);
// Get list of extracted files for ($i = 0; $i < $zip->numFiles; $i++) { $extractedFiles[] = $zip->getNameIndex($i); }
// Close the ZIP archive $zip->close();
return [ 'success' => true, 'files' => $extractedFiles ]; } else { // Extraction failed, get error message switch ($result) { case ZipArchive::ER_INCONS: $error = 'Inconsistent ZIP archive.'; break; case ZipArchive::ER_MEMORY: $error = 'Memory allocation failure.'; break; case ZipArchive::ER_NOENT: $error = 'ZIP file not found.'; break; case ZipArchive::ER_NOZIP: $error = 'Not a ZIP archive.'; break; case ZipArchive::ER_OPEN: $error = 'Could not open file.'; break; case ZipArchive::ER_READ: $error = 'Read error.'; break; case ZipArchive::ER_SEEK: $error = 'Seek error.'; break; default: $error = 'Unknown error (code: ' . $result . ').'; break; }
return [ 'success' => false, 'error' => $error ]; }}
Technical implementation features:
- Uses the native PHP
ZipArchive
class for optimal performance - Implements granular error handling with specific error codes from the
ZipArchive
class - Builds a comprehensive list of extracted files for verification
- Follows proper resource management by explicitly closing the ZIP archive
- Returns a structured array with consistent keys for result processing
4. Security Implementation
// Handle extraction request$extractionResult = null;if (isset($_POST['extract']) && isset($_POST['zipFile'])) { $zipFile = $_POST['zipFile'];
// Basic security check to prevent directory traversal if (strpos($zipFile, '../') !== false || strpos($zipFile, '..\\') !== false) { $extractionResult = [ 'success' => false, 'error' => 'Invalid file path.' ]; } else { $extractionResult = extractZip($zipFile); }}
Security mechanisms:
- Validates required parameters before processing
- Implements directory traversal protection by checking for path manipulation sequences
- Accounts for both Unix (
../
) and Windows (..\
) path separators - Returns structured error response on security violations
- Does not expose system paths in error messages
Technical Limitations
- The script extracts all files to the current directory without a configurable destination
- Directory traversal protection only checks for direct path manipulation, not encoded variants
- Lacks authentication mechanisms for restricting access to authorized users
- Does not implement file type verification beyond the
.zip
extension
Script 2: Folder Deletion Tool (delete-folder.php
)
Technical Architecture
delete-folder.php
provides a specialized interface for recursively deleting directory structures. The script implements:
- Base directory restriction for security
- Directory scanning and listing
- Multi-step confirmation process
- Recursive directory deletion with error handling
- User feedback mechanisms
Code Analysis
1. Base Directory Configuration
// Base directory - set this to limit which directories can be accessed// For safety, default to the current directory$baseDir = realpath(dirname(__FILE__));
Technical implementation notes:
- Uses
realpath()
to resolve the absolute canonical path - Prevents symbolic link attacks by resolving to the real filesystem path
- Sets a restrictive default (current directory) for maximum security
2. Directory Recursion Algorithm
function deleteDirectory($dir) { if (!file_exists($dir)) { return true; }
if (!is_dir($dir)) { return unlink($dir); }
$files = array_diff(scandir($dir), ['.', '..']);
foreach ($files as $file) { $path = $dir . DIRECTORY_SEPARATOR . $file;
if (is_dir($path)) { deleteDirectory($path); } else { unlink($path); } }
return rmdir($dir);}
Algorithm characteristics:
- Implements a depth-first traversal of the directory tree
- Uses recursive function calls for subdirectory processing
- Handles edge cases (non-existent directories, files misidentified as directories)
- Uses
DIRECTORY_SEPARATOR
constant for cross-platform compatibility - Returns boolean success indicators for error tracking
3. Path Security Validation
// Security check: Make sure the folder is within the base directory$realSelectedPath = realpath($selectedFolder);
if ($realSelectedPath === false) { $message = "Error: The selected folder does not exist."; $messageType = "error";} elseif (strpos($realSelectedPath, $baseDir) !== 0) { $message = "Error: You can only delete folders within the allowed directory."; $messageType = "error";} else { // Deletion code here}
Security mechanisms:
- Uses
realpath()
to resolve symbolic links and normalize paths - Validates that the resolved path exists in the filesystem
- Ensures the target path is a subdirectory of the base directory
- Uses string position comparison (
strpos() === 0
) to verify directory hierarchy - Provides informative error messages without exposing system paths
4. Multi-step Confirmation
// Confirmation stepif (!isset($_POST['confirm'])) { $message = "Please confirm deletion of the folder: " . htmlspecialchars($folderDeleted); $messageType = "warning";} else { // Attempt deletion try { if (deleteDirectory($realSelectedPath)) { $message = "Successfully deleted folder: " . htmlspecialchars($folderDeleted); $messageType = "success"; } else { $message = "Failed to delete folder: " . htmlspecialchars($folderDeleted); $messageType = "error"; } } catch (Exception $e) { $message = "Error: " . $e->getMessage(); $messageType = "error"; }}
Implementation features:
- Two-step confirmation process for destructive operations
- Exception handling for unexpected errors during deletion
- Consistent messaging format for different result types
- Uses
htmlspecialchars()
to prevent XSS vulnerabilities in output
Script 3: Comprehensive File and Folder Management (delete-script.php
)
Technical Architecture
delete-script.php
provides a unified interface for managing both files and directories. The script:
- Scans directories for all content (files and folders)
- Presents a table-based interface with type indicators and file sizes
- Provides individual delete buttons for each item
- Implements the same security features as the folder deletion tool
- Adds file type and size metadata for improved user experience
Code Analysis
1. Enhanced File and Folder Discovery
function getFilesAndFolders($directory) { $items = [];
if ($handle = opendir($directory)) { while (false !== ($entry = readdir($handle))) { if ($entry != "." && $entry != "..") { $fullPath = $directory . DIRECTORY_SEPARATOR . $entry;
// Store relative path to make it easier to display $relativePath = str_replace($GLOBALS['baseDir'] . DIRECTORY_SEPARATOR, '', $fullPath);
// Add type indicator $type = is_dir($fullPath) ? 'folder' : 'file';
// For files, get extension and size $extension = ''; $size = ''; if ($type === 'file') { $extension = strtolower(pathinfo($entry, PATHINFO_EXTENSION)); $size = filesize($fullPath); // Format file size if ($size < 1024) { $size = $size . ' B'; } elseif ($size < 1048576) { $size = round($size / 1024, 2) . ' KB'; } else { $size = round($size / 1048576, 2) . ' MB'; } }
$items[] = [ 'path' => $fullPath, 'name' => $relativePath ?: $entry, 'type' => $type, 'extension' => $extension, 'size' => $size ]; } } closedir($handle); }
// Sort items: folders first, then files, both alphabetically usort($items, function($a, $b) { if ($a['type'] === $b['type']) { return strcasecmp($a['name'], $b['name']); } return ($a['type'] === 'folder') ? -1 : 1; });
return $items;}
Technical implementation features:
- Collects comprehensive metadata for each filesystem item
- Implements human-readable file size formatting with appropriate units
- Uses case-insensitive string comparison for alphabetical sorting
- Prioritizes directories over files in the listing order
- Stores both absolute paths (for operations) and relative paths (for display)
- Uses global variable access for configuration consistency
2. Unified Deletion Handler
// Attempt deletiontry { if ($itemType === "folder") { $result = deleteDirectory($realSelectedPath); } else { $result = unlink($realSelectedPath); }
if ($result) { $message = "Successfully deleted " . $itemType . ": " . htmlspecialchars($itemDeleted); $messageType = "success"; } else { $message = "Failed to delete " . $itemType . ": " . htmlspecialchars($itemDeleted); $messageType = "error"; }} catch (Exception $e) { $message = "Error: " . $e->getMessage(); $messageType = "error";}
Implementation characteristics:
- Conditional logic based on item type
- Uses native
unlink()
function for file deletion - Reuses the recursive
deleteDirectory()
function for directories - Consistent error handling for both operation types
- Maintains type-specific messaging for user feedback
3. Enhanced User Interface Elements
<table class="file-list"> <thead> <tr> <th>Type</th> <th>Name</th> <th>Size</th> <th>Action</th> </tr> </thead> <tbody> <?php foreach ($items as $item): ?> <tr> <td> <?php if ($item['type'] === 'folder'): ?> <span class="folder-icon">ðŸ“</span> Folder <?php elseif ($item['extension'] === 'zip'): ?> <span class="zip-icon">🗜ï¸</span> ZIP File <?php else: ?> <span class="file-icon">📄</span> File <?php endif; ?> </td> <td><?php echo htmlspecialchars($item['name']); ?></td> <td><?php echo $item['size']; ?></td> <td> <form method="post" onsubmit="return confirm('Are you sure you want to delete this <?php echo $item['type']; ?>?');"> <input type="hidden" name="item" value="<?php echo htmlspecialchars($item['path']); ?>"> <button type="submit" name="delete" class="delete-btn">Delete</button> </form> </td> </tr> <?php endforeach; ?> </tbody></table>
UI technical features:
- Table-based layout for structured data presentation
- Visual icon differentiation for different file types
- Client-side JavaScript confirmation for deletion operations
- Form-based action buttons for each item
- Hidden input fields for maintaining state between requests
- XSS protection on all dynamically generated content
Technical Applications and Use Cases
1. Web Application Deployment
These tools are valuable in web application deployment scenarios:
- Package Extraction:
extract-zip.php
can unpack deployment packages directly on the server - Cleanup Operations:
delete-folder.php
can remove outdated releases or temporary installation files - Version Management:
delete-script.php
provides a unified interface for managing deployment artifacts
Example workflow:
- Upload deployment package:
deployment-v1.2.3.zip
to your server - Access
http://example-domain.com/extract-zip.php
in your browser - Select
deployment-v1.2.3.zip
from the dropdown and click “Extract ZIP File” - Verify the extracted files in the success screen
- If needed, use
http://example-domain.com/delete-script.php
to clean up the original ZIP file
Implementation approach:
// Example addition to extract-zip.php for deployment versioning$deploymentDirectory = 'releases/v' . date('Y.m.d-His');mkdir($deploymentDirectory, 0755, true);$zip->extractTo($deploymentDirectory);
2. Content Management Systems
For CMS applications, these scripts provide:
- Plugin/Theme Installation: Extract plugin or theme ZIP packages to the appropriate directories
- Media Management: Clean up unused media directories
- Bulk Content Operations: Delete generated files and directories for expired content
Example workflow:
- Download a new theme or plugin as a ZIP file from the developer’s site
- Upload the ZIP file to your server (via FTP or other means)
- Navigate to
http://your-cms-site.com/extract-zip.php
in your browser - Extract the theme directly to your web root
- Access
http://your-cms-site.com/delete-folder.php
to remove any deprecated themes
Implementation approach:
// Example modification for CMS plugin installationfunction extractPlugin($zipFile, $pluginDirectory) { $zip = new ZipArchive(); $result = $zip->open($zipFile);
if ($result === TRUE) { // Create target directory if it doesn't exist if (!file_exists($pluginDirectory)) { mkdir($pluginDirectory, 0755, true); }
$zip->extractTo($pluginDirectory); $zip->close(); return true; } return false;}
3. Server Administration
For server administrators, these tools provide:
- Remote File Management: Browser-based alternative to FTP or SSH access
- Disk Space Management: Identify and remove large directories consuming storage
- Maintenance Operations: Extract updates or clean up log directories
Example workflow:
- Upload system updates as a ZIP file to your server
- Browse to
http://server-ip/admin/extract-zip.php
- Extract the updates package to apply changes
- Navigate to
http://server-ip/admin/delete-script.php
to view all files and directories - Clean up temporary directories and outdated backup files
4. Secure File Hosting
For file hosting applications, these tools can be adapted to:
- User Upload Processing: Extract user-uploaded ZIP files to their storage areas
- Storage Quota Management: Delete user files to maintain storage quotas
- Content Organization: Provide structured file management capabilities to end users
Implementation approach:
// Example modification for multi-tenant file hosting$userDirectory = 'users/' . $userId . '/files/';$baseDir = realpath($userDirectory); // Restrict operations to user's directory
Technical Enhancements for Production Use
For production deployment, these scripts should be enhanced with:
1. Authentication & Authorization
Note: As currently implemented, these scripts are immediately accessible to anyone who knows the URL. For production environments, it’s critical to add authentication before deployment.
// Example basic authentication implementationsession_start();if (!isset($_SESSION['authenticated']) || $_SESSION['authenticated'] !== true) { // Redirect to login page or show authentication form header('Location: login.php'); exit;}
2. Enhanced Security Validation
// More robust path traversal preventionfunction isPathSafe($path) { $realPath = realpath($path); $basePath = realpath($GLOBALS['baseDir']);
// Check if path exists and is within base directory if ($realPath === false || strpos($realPath, $basePath) !== 0) { return false; }
// Check for encoded path traversal attempts $decodedPath = urldecode($path); if ($path !== $decodedPath && strpos($decodedPath, '..') !== false) { return false; }
return true;}
3. Logging and Audit Trails
// Example logging implementationfunction logOperation($operation, $path, $result, $userId) { $logEntry = [ 'timestamp' => date('Y-m-d H:i:s'), 'operation' => $operation, 'path' => $path, 'result' => $result ? 'success' : 'failure', 'user' => $userId, 'ip' => $_SERVER['REMOTE_ADDR'] ];
file_put_contents( 'file_operations.log', json_encode($logEntry) . PHP_EOL, FILE_APPEND );}
4. Configurable Base Directories
// Configuration file approach$config = include 'config.php';$baseDir = realpath($config['base_directory']);
// Or environment variable approach$baseDir = realpath(getenv('FILE_MANAGER_BASE_DIR') ?: dirname(__FILE__));
Installation Instructions
To implement these tools on your server:
-
Download or create the three PHP files with the provided code:
extract-zip.php
delete-folder.php
delete-script.php
-
Upload the files to your web server using FTP or your preferred file transfer method
-
Set appropriate permissions:
Terminal window chmod 644 extract-zip.php delete-folder.php delete-script.php -
Access the tools through your web browser:
http://your-domain.com/extract-zip.phphttp://your-domain.com/delete-folder.phphttp://your-domain.com/delete-script.php -
For local development with PHP’s built-in server:
Terminal window php -S localhost:8000Then access:
http://localhost:8000/extract-zip.php
Conclusion
These PHP file management tools demonstrate effective implementation of server-side file operations. The key technical strengths include:
- Security-conscious design: Path validation, input sanitization, and restricted operations
- Error handling: Comprehensive error detection and user-friendly messaging
- Resource management: Proper handling of file handles and memory-intensive operations
- Cross-platform compatibility: Using PHP constants and functions that work across operating systems
- Browser-based accessibility: Immediate access via standard HTTP URLs without additional configuration
While suitable for administrative use in protected environments, production deployment should include the enhancements outlined above, particularly authentication mechanisms and more robust security validation.
These utilities serve as both practical tools for server management and educational examples of handling filesystem operations securely in PHP applications.
Additional Resources
Files for Download
- GitHub Repository: PHP File Management Tools
Any Questions?
Contact me on any of my communication channels: